First let's use an image and specify it as a project resource. [By the way this is the image of great historical site at Lahore called Minar-e-Pakistan. So if you visit Lahore, don't forget to see this.]
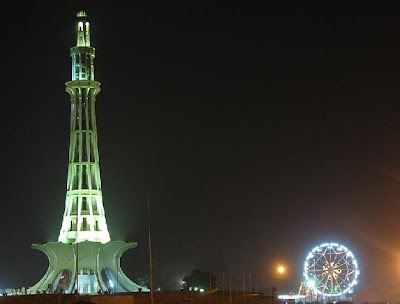
Let's save this image as Project Resource. To do that we can copy and paste the image or add as existing item in Resource section of Project. Just take the properties of the project and go to the Resource tab.
This is interesting to note that the images exposed by Project properties are System.Drawing.Bitmap type. So we need to define a converter to convert it to BitmapImage. So let's define an IValueConverter.
public class WPFBitmapConverter : IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
MemoryStream ms = new MemoryStream();
((System.Drawing.Bitmap)value).Save(ms, System.Drawing.Imaging.ImageFormat.Bmp);
BitmapImage image = new BitmapImage();
image.BeginInit();
ms.Seek(0, SeekOrigin.Begin);
image.StreamSource = ms;
image.EndInit();
return image;
}
public object ConvertBack(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
throw new NotImplementedException();
}
#endregion
}
In Convert() method, we have used a System.IO.MemoryStream to convert from System.Drawing.Bitmap to System.Windows.Media.Imaging.BitmapImage.
Now we add a Window named WindowImageProjectResource.xaml. We are initializing the converter in the Resources section of the Window. We are using this as the image converter during binding of the image with the image in the project resource.
<Window x:Class="WPFComboBoxEditable.WindowImageProjectResource"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:ProjectProperties="clr-namespace:WPFComboBoxEditable.Properties"
xmlns:local="clr-namespace:WPFComboBoxEditable"
Title="WindowImageProjectResource" Height="368" Width="616">
<Window.Resources>
<local:WPFBitmapConverter x:Key="ImageConverter" />
</Window.Resources>
<Grid>
<Image Margin="2,3,4,4" Name="image1" Stretch="Fill" >
<Image.Source>
<Binding Source="{x:Static ProjectProperties:Resources.Minar}"
Converter="{StaticResource ImageConverter}" />
</Image.Source>
</Image>
</Grid>
</Window>
As we run the application, the window is displayed with image (Minar) as follows:
2 comments:
This works very well.
I could not put application resources images into an image
control from code but I copied this class and in my code
I call the Convert method.
If you cast the result to an ImageSource - i.e.,
(ImagesSource)Convert
(Properties.Resources.ImgName,null,null,null)
- you can pop it into the Source property very easily.
needed to also use PublicResXFileCodeGenerator
http://stackoverflow.com/questions/19586401/error-in-binding-resource-string-with-a-view-in-wpf
Post a Comment