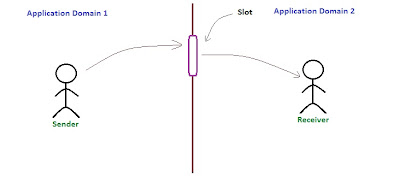
Let's define a Window to demonstrate the usage of AppDomain's slot to send and receive message to the Application Domain.
<Window x:Class="AppDomain_Slots.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Main Window" Height="350" Width="525">
<Grid>
<Label Content="Message" Height="27" HorizontalAlignment="Left" Margin="8,0,0,0"
Name="labelMessage" VerticalAlignment="Top" Width="96" FontWeight="Bold" />
<Button Content="Send Message" Height="34" HorizontalAlignment="Left" Margin="12,265,0,0"
Name="btnSendMessage" VerticalAlignment="Top" Width="150" Click="btnSendMessage_Click" />
<Button Content="Receive Message" Height="33" HorizontalAlignment="Left" Margin="337,265,0,0"
Name="btnReceiveMessage" VerticalAlignment="Top" Width="154" Click="btnReceiveMessage_Click" />
<TextBox Height="231" HorizontalAlignment="Left" Margin="8,23,0,0"
Name="textBoxMessage" VerticalAlignment="Top" Width="481" />
</Grid>
</Window>
Instantiating Application Domain:
Let's create a new application domain appDomain. We assign it a friendly name MyNewDomain.
AppDomain appDomain;
public MainWindow()
{
InitializeComponent();
appDomain = AppDomain.CreateDomain("MyNewDomain");
}
Sending message to Application Domain:
Let's write some message in a named slot of the application domain. The name of the slot is MyMessage. We will be using the text entered in the Message text box.
private void btnSendMessage_Click(object sender, RoutedEventArgs e)
{
string message = this.textBoxMessage.Text;
appDomain.SetData("MyMessage", message);
}
Receiving message in Application Domain:
Now let's receive this message in the other application domain. We would be getting this message in a callback executed in the other application domain using DoCallBack method. Since displayMessage is a static method, we don't need to serialize it.
private void btnReceiveMessage_Click(object sender, RoutedEventArgs e)
{
appDomain.DoCallBack(displayMessage);
}
static void displayMessage()
{
string message = (string)AppDomain.CurrentDomain.GetData("MyMessage");
MessageBox.Show(string.Format("Domain: {0}, Message: {1}",
AppDomain.CurrentDomain.FriendlyName,
message));
}
Let's enter some text in the Text box and hit Send Message button. This would write message to the slot MyMessage in MyNewDomain application domain.
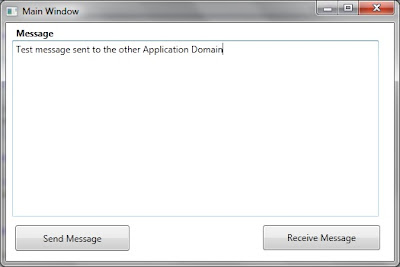
Now click Receive Message button. This would get data as in the slot and show it in the message box as follows:
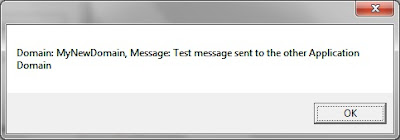
Download:
No comments:
Post a Comment